效果:
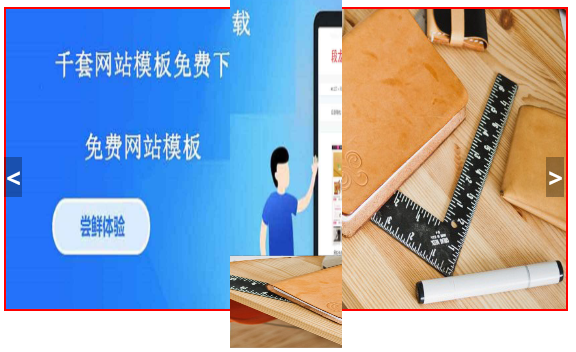
完整demo代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.js"></script>
<style>
.container {
position: relative;
width: 560px;
height: 300px;
}
.container ul {
width: 560px;
height: 300px;
border: 2px solid red;
list-style-type: none;
margin: 0;
padding: 0;
}
.container ul li {
position: relative;
float: left;
width: 112px;
height: 300px;
transform-style: preserve-3d;
transition: all 0.5s;
}
.container ul li span {
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%
}
.container ul li span:nth-child(1) {
background: yellow;
transform: translateZ(150px);
}
.container ul li span:nth-child(2) {
background: pink;
transform: translateY(-150px) rotateX(90deg);
}
.container ul li span:nth-child(3) {
background: orange;
transform: translateZ(-150px) rotateX(180deg);
}
.container ul li span:nth-child(4) {
background: blue;
transform: translateY(150px) rotateX(270deg);
}
.container ul li span:nth-child(1) {
background: url(http:
background-size: 560px 300px;
}
.container ul li span:nth-child(2) {
background: url(http:
background-size: 560px 300px;
}
.container ul li span:nth-child(3) {
background: url(http:
background-size: 560px 300px;
}
.container ul li span:nth-child(4) {
background: url(http:
background-size: 560px 300px;
}
.container ul li:nth-child(1) span {
background-position: 0px 0px;
}
.container ul li:nth-child(2) span {
background-position: -112px 0px;
}
.container ul li:nth-child(3) span {
background-position: -224px 0px;
}
.container ul li:nth-child(4) span {
background-position: -336px 0px;
}
.container ul li:nth-child(5) span {
background-position: -448px 0px;
}
.container span.left,
.container span.right {
position: absolute;
top: 50%;
background: rgba(0, 0, 0, 0.3);
width: 18px;
height: 40px;
font-size: 25px;
font-weight: bold;
color: white;
text-align: center;
line-height: 40px;
cursor: pointer;
}
.container span.left {
left: 0;
}
.container span.right {
right: 0;
}
</style>
</head>
<body>
<div class="container">
<ul>
<li>
<span></span>
<span></span>
<span></span>
<span></span>
</li>
<li>
<span></span>
<span></span>
<span></span>
<span></span>
</li>
<li>
<span></span>
<span></span>
<span></span>
<span></span>
</li>
<li>
<span></span>
<span></span>
<span></span>
<span></span>
</li>
<li>
<span></span>
<span></span>
<span></span>
<span></span>
</li>
</ul>
<span class="left">
<</span> <span class="right">>
</span>
</div>
</body>
<script>
$(function() {
var allowClick = true;
var seq = 0;
$("ul>li").each(function(index, item) {
var delay_time = index * 0.25;
$(item).css({
"transition-delay": delay_time + "s"
});
});
$("ul>li:last-child").on('transitionend', function() {
allowClick = true;
});
$("span.left").on('click', function() {
if (allowClick == false) {
return;
}
allowClick = false;
seq--;
var angle = -seq * 90;
$("ul>li").css({
"transform": "rotateX(" + angle + "deg)"
});
});
$("span.right").on('click', function() {
if (allowClick == false) {
return;
}
allowClick = false;
seq++;
var angle = -seq * 90;
$("ul>li").css({
"transform": "rotateX(" + angle + "deg)"
});
});
});
</script>
</html>